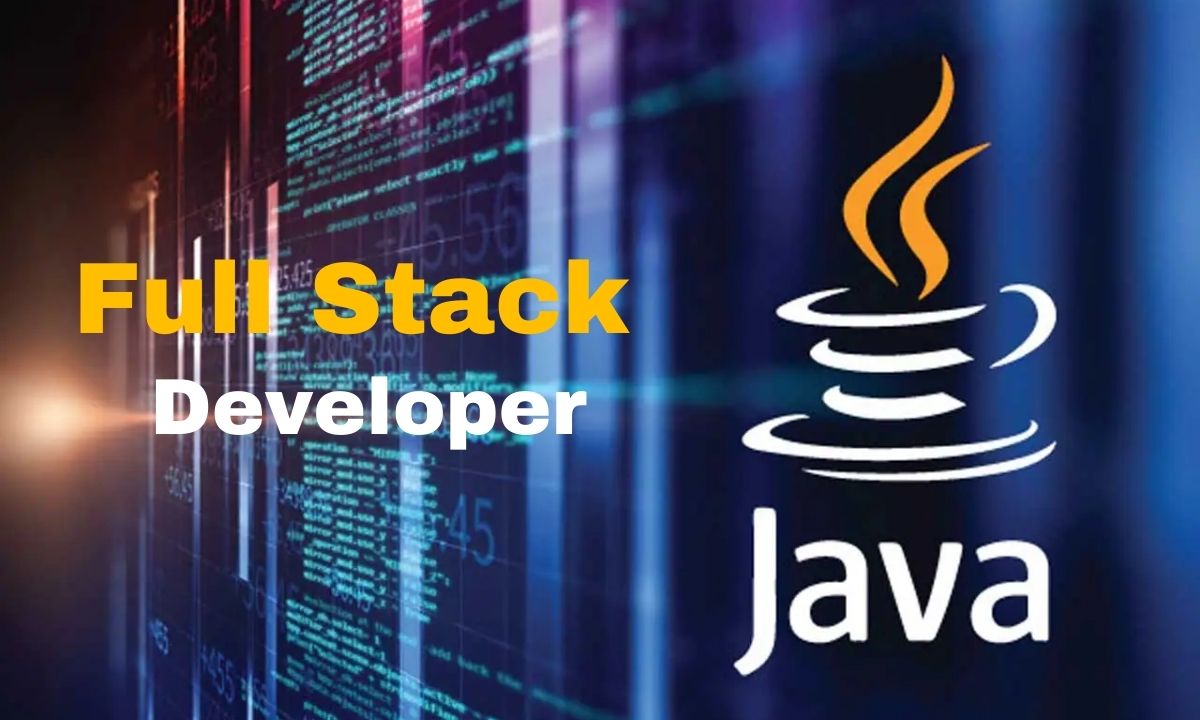
Step-by-Step Guide to Building Web Applications
Building web applications involves several steps, from planning and design to development and deployment. Here’s a step-by-step guide to help you navigate the process:
Define Requirements: Understand the purpose of your web application. What problem does it solve? Who are the users? What features do they need?
Wireframing and Prototyping: Create wireframes and prototypes to visualize the layout and user interface of your application. Tools like Figma, Sketch, or Adobe XD can be helpful.
Choose a Tech Stack: Select the appropriate technologies for your project, including programming languages, frameworks, libraries, and databases. Popular choices include JavaScript (with frameworks like React, Angular, or Vue.js), Python (with Django or Flask), Ruby on Rails, and many others.
Set Up Development Environment: Install necessary software and tools for development, including code editors, version control systems (like Git), and development servers.
Backend Development: Start building the backend of your application, which includes server-side logic, databases, and APIs. Define your data models, create RESTful or GraphQL APIs, and implement business logic.
Frontend Development: Develop the frontend of your application using HTML, CSS, and JavaScript. Use frameworks or libraries to streamline development and create interactive user interfaces.
Integration: Integrate the backend and frontend components of your application. Make API calls from the frontend to fetch data from the backend and display it to users.
Testing: Write tests to ensure the reliability, security, and performance of your application. Use automated testing frameworks for both frontend and backend testing.
Deployment: Choose a hosting provider and deploy your application to a production environment. Common choices include platforms like Heroku, AWS, Google Cloud, or DigitalOcean. Configure servers, databases, and domain settings.
Monitoring and Maintenance: Set up monitoring tools to track the performance and usage of your application. Monitor server health, application errors, and user behavior. Regularly update your application to fix bugs, add new features, and improve performance.
Security: Implement security best practices to protect your application from common threats like SQL injection, cross-site scripting (XSS), and cross-site request forgery (CSRF). Use HTTPS, authentication, and authorization mechanisms to safeguard user data.
Scalability: Design your application to scale as user traffic grows. Use techniques like caching, load balancing, and horizontal scaling to handle increased demand
Advantages of learning Java full stack?
Versatility: Java is a versatile language used for a wide range of applications, from web development to mobile apps, enterprise systems, and more. By learning Java full stack, you gain skills that are applicable across various domains.
Market Demand: Java has been a dominant force in the software industry for decades, and there’s still high demand for Java developers. Many companies, especially large enterprises, rely on Java for their backend systems, making Java full stack developers highly sought after.
Robust Ecosystem: Java has a robust ecosystem of frameworks, libraries, and tools for both backend and frontend development. Frameworks like Spring Boot, Hibernate, and Apache Struts simplify backend development, while libraries like JavaServer Faces (JSF) and Apache Wicket are popular for frontend development.
Job Opportunities: Learning Java full stack can open up a wide range of job opportunities in the software industry. Whether you’re interested in web development, enterprise software, or mobile apps, Java skills are highly valued by employers.
Scalability and Performance: Java is known for its scalability and performance, making it suitable for building high-traffic web applications and enterprise systems. Java’s robust concurrency model and memory management features contribute to its performance.
Community Support: Java has a large and active community of developers, which means plenty of resources, tutorials, and support forums are available for learning and troubleshooting. You can easily find help and guidance as you navigate your Java full stack development journey.
Cross-Platform Compatibility: Java’s “write once, run anywhere” philosophy allows you to build applications that can run on any platform with a Java Virtual Machine (JVM), including Windows, macOS, Linux, and even mobile devices.
Security: Java has built-in security features, such as sandboxing and automatic memory management, that help prevent common security vulnerabilities like buffer overflows and memory leaks.
Types of Java
Java is a versatile programming language used in various domains, and there are different “types” or flavors of Java, each tailored to specific purposes. Here are some common types of Java:
Java SE (Standard Edition): Java SE is the core Java platform used for developing desktop applications, command-line tools, and standalone applications. It provides essential libraries and APIs for general-purpose programming.
Java EE (Enterprise Edition): Java EE, now known as Jakarta EE, is a set of specifications and APIs for building enterprise-level applications, particularly web applications and services. It includes features like servlets, JavaServer Pages (JSP), Enterprise JavaBeans (EJB), Java Persistence API (JPA), and more.
Java ME (Micro Edition): Java ME is a version of Java optimized for embedded systems, mobile devices, and other resource-constrained environments. It provides a subset of Java SE features tailored for devices with limited memory, processing power, and display capabilities.
Android Java: Although not an official type of Java, Android development primarily uses Java (along with Kotlin) for building mobile applications on the Android platform. Android Java development involves using the Android SDK, which provides additional libraries and APIs specific to Android app development.
JavaFX: JavaFX is a platform for building rich client applications, including desktop and embedded applications with modern UIs. It provides a set of Java libraries and APIs for creating interactive graphical user interfaces (GUIs), multimedia applications, and more.
Spring Framework: Spring is a popular open-source framework for building enterprise Java applications. It provides comprehensive infrastructure support for developing Java applications, including dependency injection, aspect-oriented programming, data access, and more. Spring Boot, a part of the Spring ecosystem, simplifies the setup and development of Java-based web applications.
Microservices Frameworks: Various frameworks and libraries, such as Spring Boot, Micronaut, and Quarkus, are used for building microservices-based applications in Java. These frameworks offer features like lightweight containers, fast startup times, and support for cloud-native development.
Other Frameworks and Libraries: There are numerous other Java frameworks and libraries available for specific purposes, such as Hibernate for object-relational mapping (ORM), Apache Struts for web application development, Apache Camel for integration, and many more.
Learning Java offers several compelling reasons:
Versatility: Java is a versatile language that can be used for various types of software development, including web applications, mobile apps, enterprise systems, desktop applications, and more. Its flexibility makes it a valuable skill for developers working in different domains.
Market Demand: Java has been one of the most popular programming languages for decades, and there’s still high demand for Java developers in the job market. Many companies, especially large enterprises and government organizations, rely on Java for their critical systems, leading to abundant job opportunities for Java developers.
Platform Independence: Java’s “write once, run anywhere” principle allows developers to write code once and run it on any platform that supports Java, including Windows, macOS, Linux, and various mobile devices. This platform independence is achieved through the Java Virtual Machine (JVM), making Java applications highly portable.
Strong Ecosystem: Java has a robust ecosystem of frameworks, libraries, and tools that streamline development and accelerate time-to-market. Popular frameworks like Spring, Hibernate, and Apache Struts provide solutions for common development challenges, while tools like Maven and Gradle automate build processes.
Community Support: Java boasts a large and active community of developers, offering a wealth of resources, tutorials, forums, and open-source projects. Whether you’re a beginner or an experienced developer, you’ll find ample support and guidance within the Java community.
Scalability and Performance: Java’s scalability and performance make it suitable for building large-scale, high-performance applications. Java’s robust concurrency model, memory management, and performance optimization techniques ensure that Java applications can handle heavy workloads efficiently.
Security: Java was designed with security in mind, incorporating features like bytecode verification, automatic memory management, and a robust security model. Java applications are less susceptible to common security vulnerabilities like buffer overflows and memory leaks, making them a preferred choice for building secure systems.
Longevity: Java has stood the test of time and remains one of the most widely used programming languages globally. Its stability, backward compatibility, and continuous evolution through updates and new releases ensure that Java will remain relevant for years to come.
Why do we learn Java?
Wide Adoption: Java is one of the most widely adopted programming languages globally, powering a vast array of applications, from enterprise systems to mobile apps and web development. Learning Java opens up opportunities to work on diverse projects and platforms.
Versatility: Java’s versatility allows developers to build a variety of applications, including web applications, desktop software, mobile apps (Android), enterprise systems, scientific applications, and more. This flexibility ensures that Java developers have a broad range of career options.
Platform Independence: Java’s “write once, run anywhere” philosophy enables developers to write code once and run it on any platform that supports Java, thanks to the Java Virtual Machine (JVM). This platform independence simplifies development and deployment across different operating systems.
Strong Ecosystem: Java has a robust ecosystem of libraries, frameworks, tools, and resources that streamline development and accelerate the creation of complex applications. Popular frameworks like Spring, Hibernate, and Apache Struts provide solutions for common development tasks, while tools like Maven and Gradle automate build processes.
Job Opportunities: Java skills are in high demand in the job market, especially for roles in software development, application architecture, system integration, and more. Many companies, particularly large enterprises and tech giants, use Java for their core systems, creating ample job opportunities for Java developers.
Community Support: Java has a vibrant and supportive community of developers worldwide. This community provides access to resources, forums, tutorials, and open-source projects, making it easier for developers to learn, collaborate, and solve problems together.
Scalability and Performance: Java’s scalability and performance make it suitable for building high-performance, mission-critical applications. Java’s robust concurrency model, memory management, and optimization capabilities ensure that applications can handle large workloads efficiently.
Security: Java was designed with security in mind and incorporates features like bytecode verification, automatic memory management, and a strong security model. Java applications are less susceptible to common security vulnerabilities, making them a preferred choice for building secure systems.